Mastering JavaScript for Efficient Code Management and Software Development
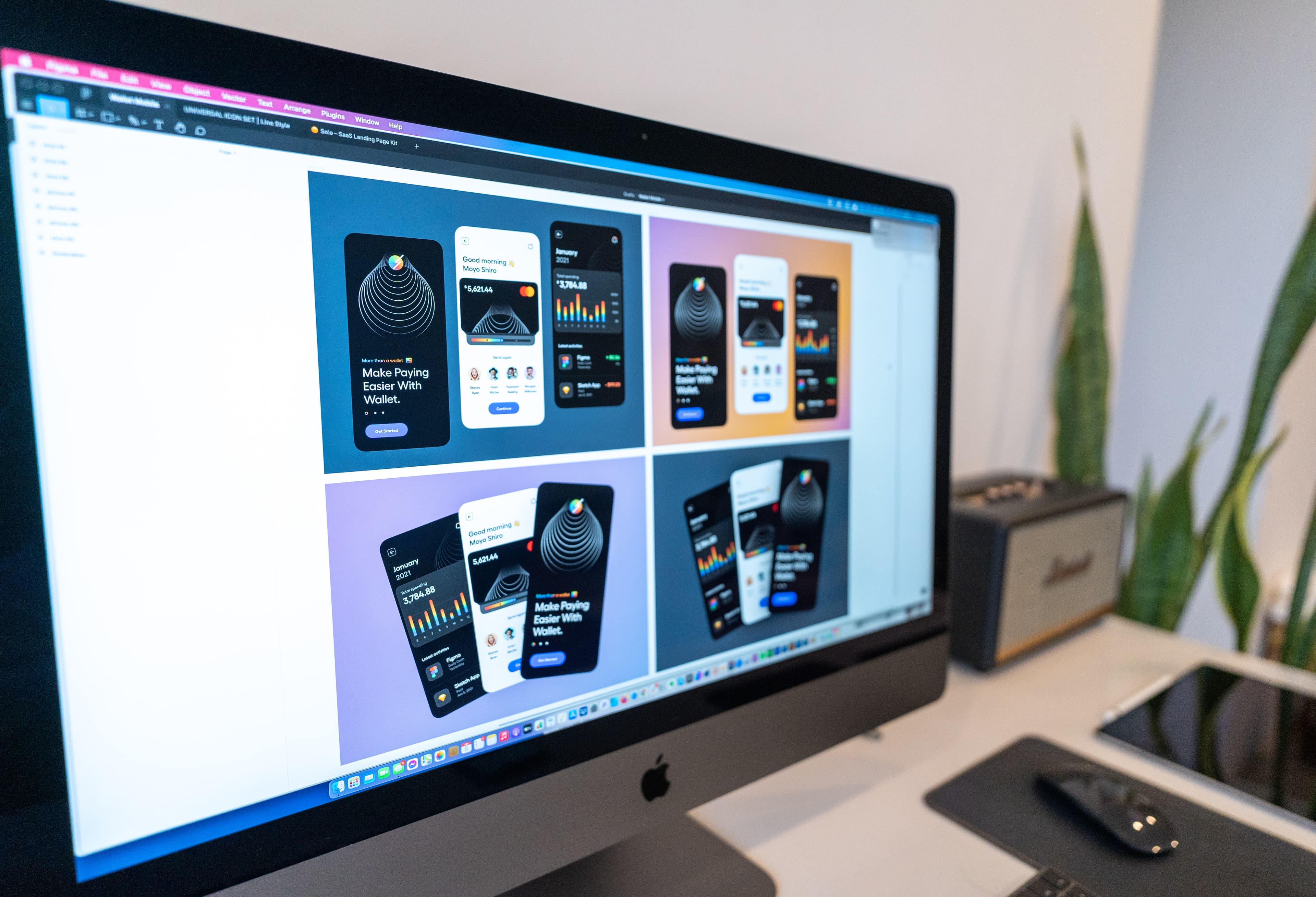
Table Of Content
- Understanding JavaScript Syntax
- Variables and Data Types
- Control Flow
- Functions
- Objects and Arrays
- Best Practices for JavaScript Development
- Code Readability
- Modularization and Code Organization
- Error handling
- Performance Optimization
- Advanced JavaScript Concepts
- Closures
- Promises and Async/Await
- Functional Programming
- Design Patterns
- Tools for Effective JavaScript Development
- Package Managers
- Build Tools
- Testing Frameworks
- Version Control Systems
- Further Learning and Resources
JavaScript has evolved into a fundamental language in the realm of web development, influencing both client-side and server-side programming.
As a programmer or developer, mastering JavaScript and understanding its principles is pivotal to achieving efficiency in code management and delivering high-quality software. In this comprehensive guide, we'll delve into crucial aspects of JavaScript, from syntax and best practices to advanced concepts and tools that facilitate streamlined software development.
With people regularly asking about how to master JavaScript:
Have you ever questioned about how to define variables properly in JavaScript? Curious about how to master the concepts in JavaScript for an aspiring web developer? We understand your concerns and are here to explore the nuances of JavaScript. Let's dive into the realm of JavaScript, where we aim to address common issues such as the basics of variables assignments, data types in JavaScript, objects and Arrays, Async and promises, control flow, error handling and many more.
Without much talk let's dive right into it!!!
Understanding JavaScript Syntax
JavaScript's syntax forms the foundation of programming in the language. Let's explore its fundamental elements.
Variables and Data Types
JavaScript is a dynamically typed language, meaning variable types are determined at runtime. Here's an example of variable declaration and usage:
let firstName = 'John'; // String
let age = 30; // Number
let isStudent = true; // Boolean
let interests = ['coding', 'reading']; // Array
let person = { firstName, age, interests }; // Object
For a deeper understanding of JavaScript data types, refer to the MDN Web Docs or w3School.
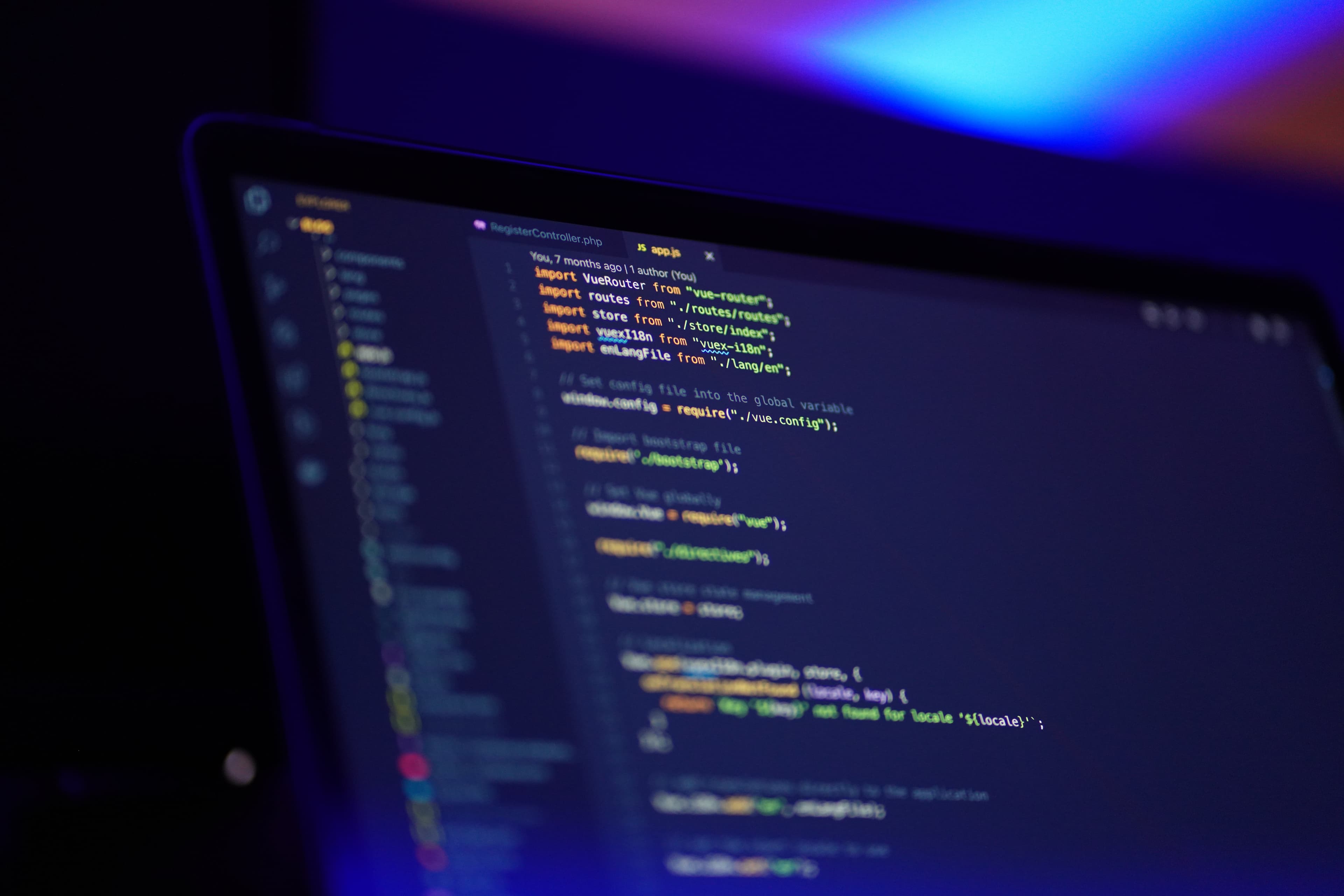
Control Flow
Control flow structures in JavaScript include conditionals (if-else, switch) and loops (for, while). Here's an example of an if-else statement:
const x = 10;
if (x > 5) {
console.log('x is greater than 5');
} else {
console.log('x is less than or equal to 5');
}
Explore more on control flow in JavaScript through MDN Docs.
Functions
Functions are essential in JavaScript for code organization and reusability. Here's a simple function that adds two numbers:
function addNumbers(a, b) {
return a + b;
}
const sum = addNumbers(5, 3);
console.log('Sum:', sum); // Output: Sum: 8
Dive deeper into functions with the MDN Docs Functions.
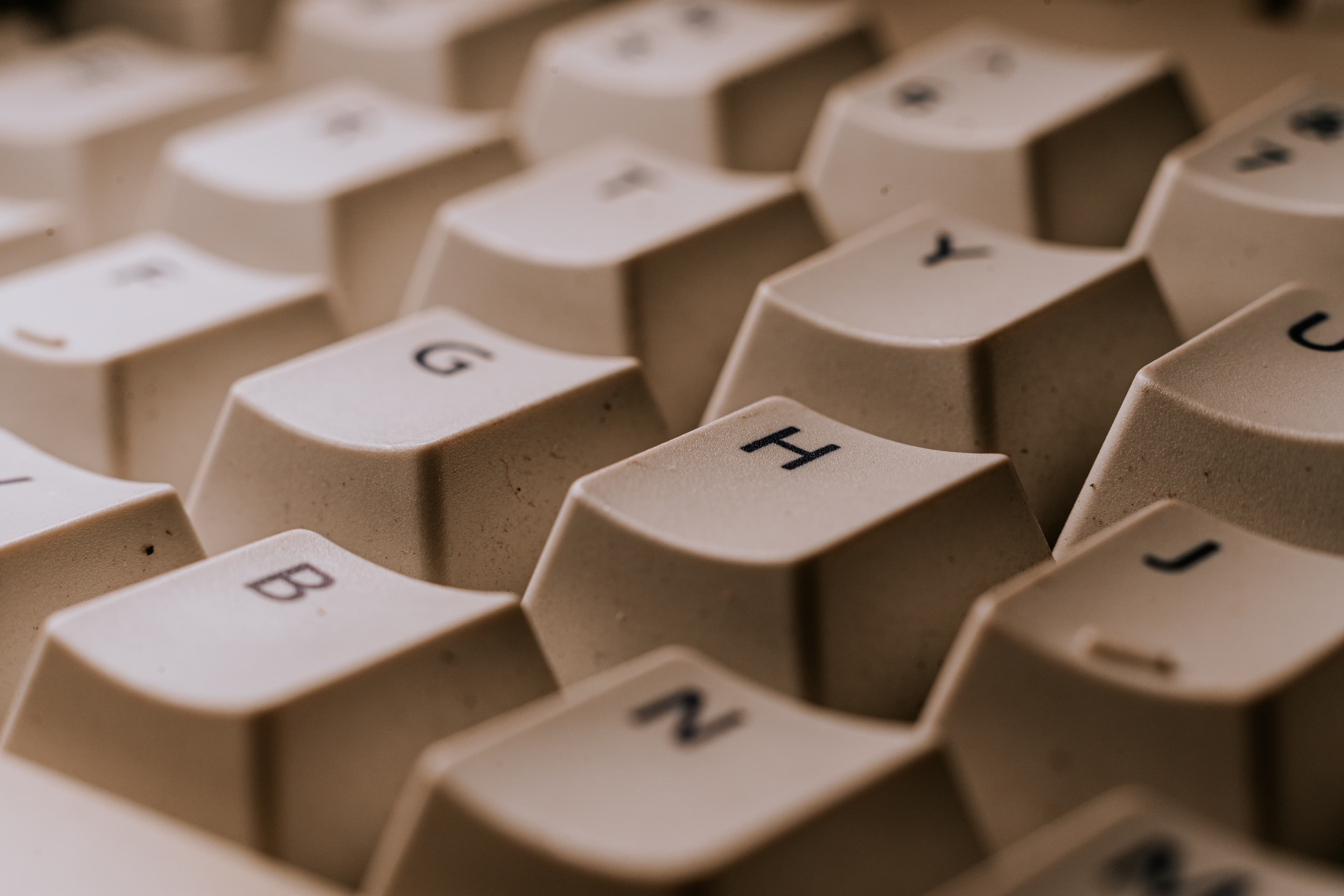
Objects and Arrays
JavaScript objects and arrays allow for structured data storage. Here's an example of each:
const person = {
firstName: 'Alice',
lastName: 'Smith'
};
const fruits = ['apple', 'banana', 'cherry'];
Learn more about objects and arrays from the Working with Objects and Working with Arrays guides.
Best Practices for JavaScript Development
To ensure efficient code management and streamlined software development, adhere to these best practices.
Code Readability
Write clear, well-formatted code with meaningful variable and function names. Use consistent indentation and follow a style guide, such as Airbnb's JavaScript Style Guide.
Modularization and Code Organization
Organize your code into modules to improve maintainability and reusability. Utilize tools like webpack to bundle and manage modules efficiently.
Error handling
Implement robust error handling to enhance the stability and reliability of your application. Use try-catch blocks for handling exceptions and providing meaningful error messages.
Performance Optimization
Optimize your JavaScript code for better performance by minimizing unnecessary operations, leveraging asynchronous programming, and optimizing DOM manipulation.
Advanced JavaScript Concepts
To master JavaScript, delve into these advanced concepts that enrich your programming skills and understanding of the language.
Closures
Closures are functions that remember the environment in which they were created, allowing them to access variables from their outer scope even after that scope has finished execution. Understanding closures is crucial for efficient memory management and creating self-contained functions.
function outer() {
const message = 'Hello, ';
function inner(name) {
console.log(message + name);
}
return inner;
}
const greet = outer();
greet('Alice'); // Output: Hello, Alice
Explore closures further in this insightful article by MDN Web docs Closures.
Promises and Async/Await
Promises and async/await are vital for handling asynchronous operations in JavaScript. Promises provide a cleaner way to handle asynchronous code, while async/await simplifies working with promises.
function asyncTask() {
return new Promise((resolve, reject) => {
setTimeout(() => {
resolve('Task completed');
}, 1000);
});
}
async function executeTask() {
try {
const result = await asyncTask();
console.log(result);
} catch (error) {
console.error('Error:', error);
}
}
executeTask();
For an in-depth understanding, visit working with promises and Working with Async.
Functional Programming
Functional programming emphasizes immutability, pure functions, and avoiding side effects. It's a paradigm that can lead to more maintainable and predictable code.
const numbers = [1, 2, 3, 4, 5];
const doubleNumbers = numbers.map(num => num * 2);
console.log(doubleNumbers); // Output: [2, 4, 6, 8, 10]
Learn about functional programming concepts in JavaScript through this guide by Functional programming.
Design Patterns
Design patterns offer solutions to common programming challenges. Understanding and applying design patterns in your codebase can enhance code maintainability and scalability.
Some commonly used design patterns in JavaScript include the Singleton, Observer, and Module patterns.
Tools for Effective JavaScript Development
To streamline your JavaScript development process, leverage these powerful tools.
Package Managers
Package managers like npm (Node Package Manager) and Yarn simplify the process of installing, managing, and updating JavaScript packages and dependencies.
Build Tools
Build tools like Webpack and Parcel help bundle, optimize, and organize your JavaScript code, making it production-ready.
Testing Frameworks
Testing frameworks like Jest and Mocha assist in writing and running tests for your JavaScript applications, ensuring code reliability and functionality.
Version Control Systems
Utilize version control systems like Git and platforms like GitHub or GitLab to collaborate, manage, and track changes in your codebase effectively.
Further Learning and Resources
To continue expanding your knowledge of JavaScript and advancing your skills as a programmer or developer, explore these valuable resources:
- Mozilla Developer Network (MDN): Comprehensive JavaScript documentation and guides.
- freeCodeCamp: Offers interactive coding challenges and tutorials on JavaScript and web development.
- Eloquent JavaScript: A book that teaches JavaScript concepts in-depth.
- JavaScript Design Patterns: A comprehensive book on design patterns in JavaScript.
By mastering JavaScript and incorporating best practices, advanced concepts, and the right tools into your workflow, you'll become a proficient developer capable of efficient code management and delivering high-quality software. Happy coding!